Hi, everyone I am glad to be back with another topic, this time I will be covering Headless CMS with flutter.
So, What is Headless CMS, or rather let's start from what is CMS itself.
CMS stands for Content Management System. It helps by controlling what content to be shown to the user, content mostly is managed by people who don’t have much knowledge about the code, so for them, it might look like just a basic form where they put in data, the data get stored in Database and then our app extracts that data from Database and displays to the user. It is useful because all the content can be managed separately and would not need to be coded manually so that even for the smallest change you won’t have to waste time searching where you put in that particular string and then change it.
Now, coming to Headless CMS
The “Headless” is the new approach towards CMS whereas in the traditional CMS it was mostly coupled with a web page but, this new approach helps to widen that horizon and makes it so that content can be displayed on any device of user's choice.
The devices are called “Heads” and since there are many devices so that means there is not a single fixed head.
This approach is better and an overall improvement over traditional CMS.
The way it works is that it provides us an API for all of our data and if we like we can use that directly in our project to show changes to the user, Hence why this approach is better than traditional CMS
What will we be using for this short demo?
For this short demo on the Headless CMS approach, we will be using StoryBlok.
It provides you an API with all your data you have entered into it.
I won’t go into too much detail over this -but all you need to do is create your own space and then define a schema and then publish that in the content and go to settings to generate your token and then get the API.
That’s basically it!
Now to get onto the coding part!
How to handle API ?
There are multiple ways of handling an API call in flutter, but the simplest would be to use the Http package from pub.dev, just paste that in the pubspec.yaml file.
http: any
That’s all you needed to do. Now to use this package we will import it as below
import 'package:http/http.dart' as http;
We have added as “http” there so that we can use its functions with ease,
Now to make an API call we can do that by making a separate function called _fetch() and make it asynchronous.
_fetch() async {
}
Now inside that function, we need to make a variable that will help us get the response from the API so we will call that response.
Its type would be of http.Response, its always better to define the types,
and to give this variable value, It will have the value of http.get(“ ”);.
Be sure to put in await before that so that we can wait for it to get the response from the API
_fetch() async {
final http.Response response = await http.get("https://api.storyblok.com/v1/cdn/stories?page=1&token=5kNqPrD6wYQRHakzUrxrGwtt");
}
Now once we are done with the API call and have gotten the response we need to check if that response was correct or not and we do that by accessing a property of http.Response type variables that are statusCode
We make a simple check that if the statusCode was 200 then no error occurred and we got the response we desired.
if(response.statusCode ==200) {
// YOUR FUNCTION BODY HERE
}
for now, once we get the API response and the statusCode is 200 let's decode it.
For that, we will use an inbuilt method called jsonDecode()
final Map<String,dynamic> json = jsonDecode(response.body);
We have accessed the body of the response we got and have gotten that body part into a json variable which is of type Map.
now we can just print the json and get all the data we require, to use the data we can use it like we use any other map
However I recommend making a Modal class for dealing with this, an example would be
class Fetch {
final name;
final created;
final published;
final alternates;
final id;
final uuid;
final content;
final slug;
final full_slug;
final default_full_slug;
final sort_by_date;
final position;
final tag_list;
final isStartPage;
final parent_id;
final meta_data;
final release_id;
final lang;
final path;
final translated_slugs;
Fetch({this.name, this.created, this.published, this.alternates, this.id,
this.uuid, this.content, this.slug, this.full_slug,
this.default_full_slug, this.sort_by_date, this.position, this.tag_list,
this.isStartPage, this.parent_id, this.meta_data, this.release_id,
this.lang, this.path, this.translated_slugs});
factory Fetch.fromFetch( map) {
return Fetch(
name: map["name"],
created: map["created_at"],
published: map["published_at"],
alternates: map["alternates"],
id: map["id"],
uuid: map["uuid"],
content: map["content"],
slug: map["slug"],
full_slug: map["full_slug"],
default_full_slug: map["default_full_slug"],
sort_by_date: map["sort_by_date"],
position: map["position"],
tag_list: map["tag_list"],
isStartPage: map["is_startpage"],
parent_id: map["parent_id"],
meta_data: map["meta_data"],
release_id: map["release_id"],
lang: map["lang"],
path: map["path"],
translated_slugs: map["translated_slugs"]
);
}
}
So we have defined all the keys in the map as a variable here and we are gonna make an object of this Modal class and use that object to show what we want from the json data.
If you want more help making a Modal class you can contact me anytime you like
Now how we provide data from json to it?
It's beyond simple really I usually do it like this
List list = json['stories'];
print(list);
list.map((i) {
Fetch fetch = Fetch.fromFetch(i);
print(fetch.id);
print("fetch name => ${fetch.name}");
}).toList();
Well in my json ‘stories’ was a key which had a List as its value so I made this so I can get the data of all list items into my modal class and print them,
You can declare another List of type Fetch and add in it the fetch instance so that you can use it whenever you like,
Example
List<Fetch> fetchList =[];
List list = json['stories'];
print(list);
list.map((i) {
Fetch fetch = Fetch.fromFetch(i);
fetchList.add(fetch);
}).toList();
Now, this makes our work much, much easier. Instead of writing the key in strings over and over again, we can simply use it like fetchList[0].name or fetchList[0].id, etc.
Now all that is left is to either bind it to a button or if you want to run it at app startup then call the function in initState();
As always you can find the full code for this in the GitHub. Hope you guys enjoyed reading through this, If you have any queries be sure to let me know, and I will try to solve them.
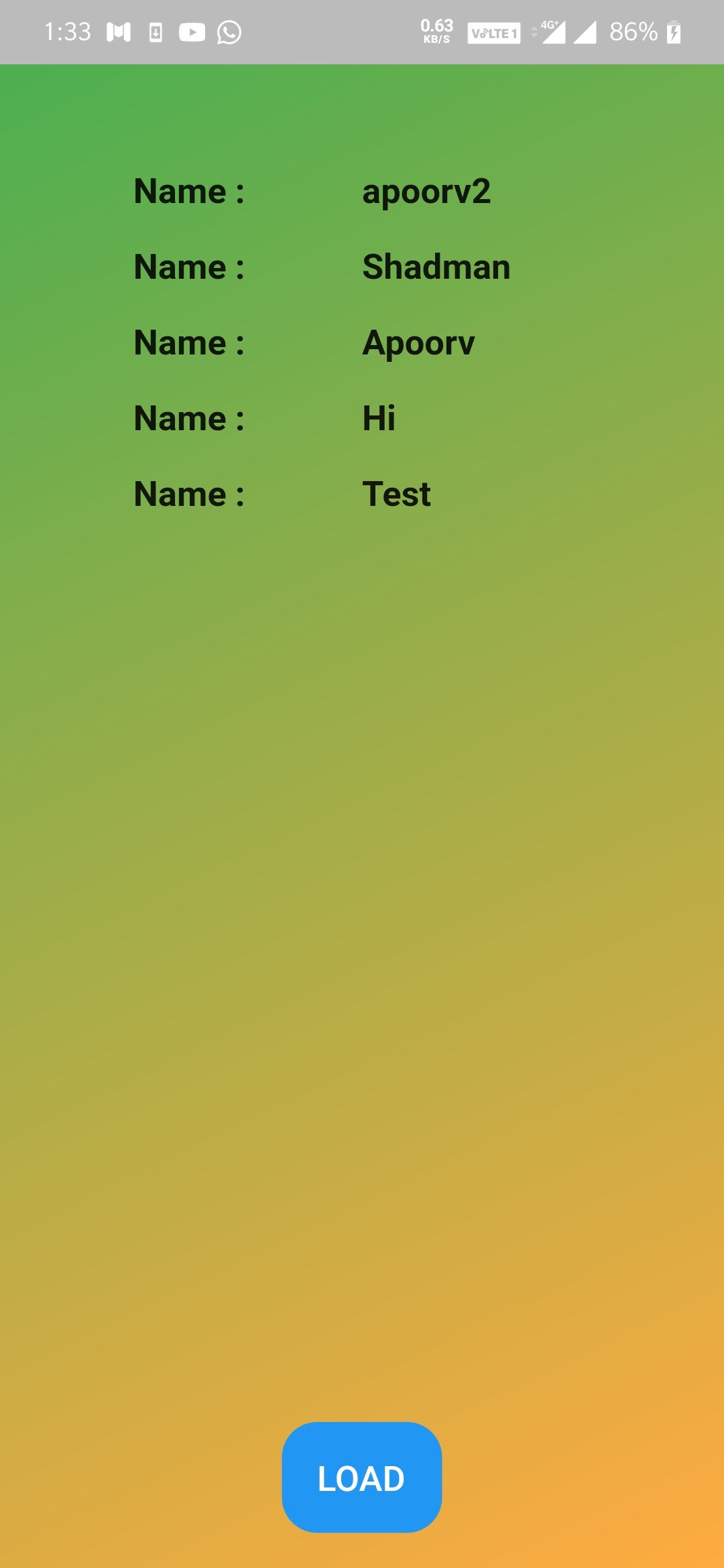
Feel free to connect with us: And read more articles from FlutterDevs.com.
FlutterDevs team of Flutter developers to build high-quality and functionally-rich apps. Hire flutter developer for your cross-platform Flutter mobile app project on an hourly or full-time basis as per your requirement! You can connect with us on Facebook, GitHub, Twitter, and LinkedIn for any flutter related queries.
We welcome feedback and hope that you share what you’re working on using #FlutterDevs. We truly enjoy seeing how you use Flutter to build beautiful, interactive web experiences.
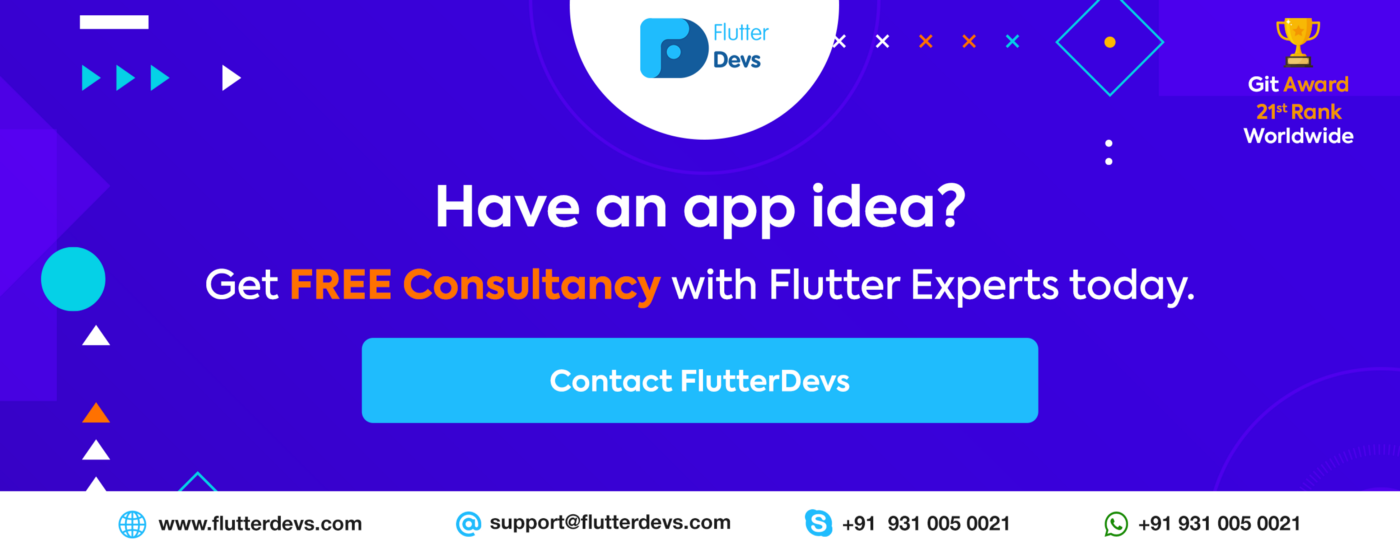